# Passwordless Authentication There are some things that need to be considered before adopting a passwordless authentication process for your system. Passwordless comes with some good points and with some potential downsides. First though ... # What is passwordless authentication Passwordless authentication is a mechanism that enables users to log in without a set password by proving that they have access to something. Passwordless is often associated with a one use code or link sent to the user via email or SMS. There are other options that can include making use of the [WebAuthn](https://www.w3.org/TR/webauthn/) standard. This post focuses on the implementations using SMS and eamil. # Why is it good Simply put, because passwords are terrible. # What's wrong with passwords We as the greater collection of web users have done a bad job with managing our passwords. We forget them, we make them easy to guess by keeping them short and basing them off personal information. If t...
Posts
Building a verify JWT function in TypeScript
- Get link
- X
- Other Apps
# Verifying an RS232 signed JWT JSON Web Tokens (JWT) are used as a way to verify the identity of the caller of an API. **The best way to verify a JWT is to use a verification library.** I wanted to have a look at some of what those libraries are doing under the hood by putting together a function that will return if a given token is valid. In this blog I'll go through what I have done to get a validation function working. To simplify things assume: - That the signing algorithm is RS232 all others are considered invalid. - That the public keys are available on a JWKS url provided to the function eg [https://klee-test.au.auth0.com/.well-known/jwks.json](https://klee-test.au.auth0.com/.well-known/jwks.json) - I only want to know if the token was signed by a key available at the above url. I wont be checking if the token has expired, if the scopes or other claims are valid. # Break up the token A JWT is made up to 3 parts. The first thing to do in validating the token is to brea...
Solving buffer.readUIntBE is not a function reading a JWKS key with NodeRSA
- Get link
- X
- Other Apps
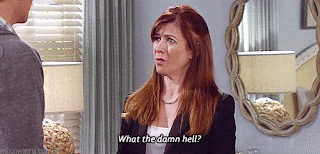
Recently while trying to read a public key in from a JWKS endpoint I was stumped by an error when importing the public key using [NodeRSA](https://github.com/rzcoder/node-rsa). My code looked like this: ```Typescript return rsa.importKey( { n: Buffer.from(jwksKey.n), e: jwksKey.e, }, "components-public" ); ``` The error was: ```bash TypeError: buffer.readUIntBE is not a function at Object.module.exports.get32IntFromBuffer (C:\dev\jwttest\node_modules\node-rsa\src\utils.js:43:27) at RSAKey.module.exports.Key.RSAKey.setPublic (C:\dev\jwttest\node_modules\node-rsa\src\libs\rsa.js:172:48) at Object.publicImport (C:\dev\jwttest\node_modules\node-rsa\src\formats\components.js:44:17) at Object.detectAndImport (C:\dev\jwttest\node_modules\node-rsa\src\formats\formats.js:65:48) at NodeRSA.module.exports.NodeRSA.importKey (C:\dev\jwttest\node_modules\node-rsa\src\NodeRSA.js:183:22) at C:\dev\jwttest\dist\jwks.js:53:20 at Gener...
Solving consent_required Auth0 SPA SDK
- Get link
- X
- Other Apps
I've been playing with the Auth0 SPA SDK https://auth0.com/docs/libraries/auth0-spa-js # What's wrong I keep running into an issue where I an error is thrown with code `consent_required` when trying to initialise the Auth0 client by calling `createAuth0Client` that is brought in from the library `import createAuth0Client from "@auth0/auth0-spa-js"`. # Why's it's going wrong Reading into it this is because the `createAuth0Client` function is calling `getTokenSilently` as part of the creation. This call fails when a user has a current session but the parameters of the authenticaion have changed to require the user accepts some updated conditions. Examples of where this is going to happen are adding or modifying the `useRefreshTokens`, `scope`, or `audience` properties passed to `createAuth0Client`. Note: This will only happen the first time that these permissions are introduced for a user. If the logged in user has previously accepted these the prompt ...
Chain of responsibility
- Get link
- X
- Other Apps
How could I explain the Chain of Responsibility pattern to myself? # What is the Chain of Responsibility? A series of classes or functions that are capable of handing certain requests.These handlers are connected in a linked list where each handler is aware of the next handler in the list, or chain. If a handler is not capable of handling the request then it calls the next providing the request. The chain may be implemented such that multiple handlers can contribute to the completion of a single request. In this the request will need to be updated before being passed to the next handler. # Why is that a good idea? * It allows handers to be added or removed with minimal change to existing code. * It allows handlers to be re-ordered without editing the handlers or making large code changes. * Allows handling logic to be decoupled from other handling logic and tested independently. # Where would you use it? * At times where the execution order is likely to be changed often or dy...
Command Object Pattern
- Get link
- X
- Other Apps
How would I explain Command Object Pattern to Myself. What is it? The Command Object Pattern to encapsulate what would have otherwise been a function call inside an object that is abstracted away behind an interface. The Command Object Pattern is a pattern of four classes: The Command Implements the Command Interface and takes a dependency on the receiver. The command encapsulates everything needed to call the receiver. The Receiver The class being on by invoking the command. The Invoker Depends on an abstract Command interface. Does not know anything about the concrete implementation of the Command. The Client High level class responsible for connecting the invoker with commands. Why is it good? The Command Object Pattern facilitates using the Open Closed Principle on the Invoker. Making the Invoker open for extension and closed for modification by providing behaviour at run time. This allows for independent testing of components and component reuse. Where woul...
Factory Method Pattern
- Get link
- X
- Other Apps
What is the Factory Method Pattern? Using a method to create a new instance of an object rather than using the new keyword to call the constructor directly. Why would you do that? This decouples the two classes by allowing the higher level class to change from creating an instance to requesting an instance of the lower level class. The advantage of decoupling the two classes by changing the nature of the relationship between the two of them. The higher level class no longer creates an instance of the lower level class. Instead this pattern means that the higher level class requests an instance of an interface.. This presents a number of advantages; * It allows for object pooling of the lower level class. Some objects may be expensive to create, consume a lot of memory, or may require that there are a limited set of them in existence. In these cases a pool of objects can be created and they can be passed out to consumers. * It allows for the higher level class ...